diff --git a/.prettierignore b/.prettierignore
index 68fdc6b..f3f941f 100644
--- a/.prettierignore
+++ b/.prettierignore
@@ -10,4 +10,5 @@ node_modules
*.lock
*.ejs
*.*-
-*.sql
\ No newline at end of file
+*.sql
+*.md
\ No newline at end of file
diff --git a/.vscode/settings.json b/.vscode/settings.json
index 3d072e3..6b09050 100644
--- a/.vscode/settings.json
+++ b/.vscode/settings.json
@@ -3,6 +3,7 @@
"prettier.eslintIntegration": false,
"cSpell.words": [
"sqlify",
+ "squel"
],
"cSpell.language": "en"
}
diff --git a/README.md b/README.md
index 2e3ed07..baf807d 100644
--- a/README.md
+++ b/README.md
@@ -1,118 +1,173 @@
# Sqlify
-Yet another SQL query builder
+
+Yet another SQL query builder.
[](https://www.npmjs.com/package/sqlify)
[](https://travis-ci.org/vajahath/sqlify)
-[](https://snyk.io/test/npm/sqlify)
-[](https://gitter.im/npm-sqlify/Lobby)
-[](https://www.npmjs.com/package/sqlify)
+[]()
> There are many sql query builders out there. But this one makes more sense to me :wink:.
-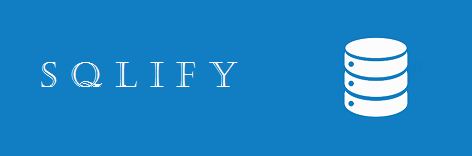
-
-
-> **Sqlify v2.3** is out! **What's new?**
-> - better error handling
-> - more squel functions (`order`, `group`)
-> - *(migrating from v1 to v2 is easier than you think. It just requires 2 mins, you bet!)*
->
[**migration guide**](#v1-to-v2-migration-guide)
-
-*:flags: This package is bound to strictly follow [Semantic Versioning](http://semver.org/).*
-
-[](https://greenkeeper.io/)
+
## Install
+
```bash
npm install --save sqlify
```
-**Read [squel](https://hiddentao.com/squel)'s documentation along with this.**
-_This package is a wrapper around [squel](https://hiddentao.com/squel)_
+## Why
-## Why?
-- Helps you to build dynamic sql queries.
-- **Example use case:** suppose, you are getting a POST request to insert some data to your SQL database.
+* This package is a wrapper around [squel](https://hiddentao.com/squel) module to make it more friendly.
+* Helps you to build dynamic sql queries.
+* **Example use case:** suppose, you are getting a POST request to insert some data to your SQL database.
You'll get the data in `req.body` as `{name: "Swat", age: 22, address: "ND"}`.
Now make the query like:
- ```js
- let resource = {
- set: req.body
- }
- sqlify(chain, resource); // done!
- ```
+ ```js
+ const resource = {
+ set: req.body
+ where: {
+ id: 5
+ }
+ }
+
+ sqlify(chain, resource); // done!
+ ```
## Examples
+
#### SELECT
+
```js
-const sql = require('sqlify').squel;
-const sqlify = require('sqlify').sqlify;
+const { squel, sqlify } = require('sqlify');
-let resource = {
+const resource = {
field: ['name', 'age', 'address'],
where: {
name: 'Swat',
- age: 22
- }
+ age: 22,
+ },
};
-let chain = sql.select().from('users');
+
+const chain = squel.select().from('users');
+
sqlify(chain, resource);
-chain.toString() // => SELECT name, age, address FROM users WHERE (name=Swat) AND (age=22)
+chain.toString();
+// => SELECT name, age, address FROM users WHERE (name=Swat) AND (age=22)
```
+##### Starter Guide For TypeScript
+
+```ts
+import { squel, sqlify, Resource } from 'sqlify'
+
+// `Resource` is type.
+const resource :Resource = {
+ field: ['name', 'age', 'address'],
+ where: {
+ name: 'Swat',
+ age: 22,
+ },
+};
+
+// ...
+```
+
+#### SELECT with a simple JOIN
+
+```js
+// ...
+
+const resource = {
+ field: ['user.*', 'hobbies.hobby', 'colors.favorite'],
+ where: {
+ name: 'Swat',
+ age: 22,
+ },
+ join: [
+ ['hobbies', null, 'hobbies.id = user.id'],
+ ['colors', null, 'colors.user_id = user.id'],
+ ];
+}
+const chain = squel.select().from('Hero');
+
+sqlify(chain, resource);
+
+chain.toString();
+
+/*
+SELECT
+ user.*,
+ hobbies.hobby,
+ colors.favorite
+FROM Hero
+ INNER JOIN hobbies
+ ON (hobbies.id = user.id)
+ INNER JOIN colors
+ ON (colors.user_id = user.id)
+WHERE (name='Swat') AND (age=22)
+*/
+```
+
+Read the JOIN section of [squel docs](https://hiddentao.com/squel/#select) for more.
+
#### INSERT
+
```js
-const sql = require('sqlify').squel;
-const sqlify = require('sqlify').sqlify;
+const { squel, sqlify } = require('sqlify');
-let resource = {
+const resource = {
set: {
name: 'Swat',
- age: 22
- }
+ age: 22,
+ },
};
-let chain = sql.insert().into('users');
+
+const chain = sql.insert().into('users');
sqlify(chain, resource);
-chain.toString() // => INSERT INTO users (name, age) VALUES ('Swat', 22)
+chain.toString();
+// => INSERT INTO users (name, age) VALUES ('Swat', 22)
```
## How?
-`sqlify` exposes a **function** and a **module** ([squel](https://www.npmjs.com/package/squel)).
+`sqlify` exposes a **function**, **module** ([squel](https://www.npmjs.com/package/squel)) and a `Resource` type (for using with TypeScript).
The function receives 2 arguments. They are:
-- `chain`
-- `resource`
+
+* `chain`
+* `resource`
#### Step 1: Require the package
-```js
-const sql = require('sqlify').squel;
-const sqlify = require('sqlify').sqlify;
-const lme = require('lme') // not necessary. For printing things out..
-...
+```js
+const { squel, sqlify } = require('sqlify');
```
#### Step 2: Initialize `chain` and `resource`
+
`chain` is an instance of [squel](https://www.npmjs.com/package/squel).
For example,
+
```js
-...
+// ...
-let chain = squel.select().from('users');
+const chain = squel.select().from('users');
-...
+// ...
```
`resource` is an object which contains the data to build the query.
+
Example:
+
```js
-...
+// ...
-let resource = {
+const resource = {
field: ['name', 'age', 'address'],
where: {
name: 'Swa',
@@ -120,100 +175,123 @@ let resource = {
}
};
-...
+// ...
```
-Where, the properties of `resource` object (in the above case, `field` and `where`) are taken from the chain function names of the [squel](https://www.npmjs.com/package/squel). Use them accordingly.
+
+Where, the properties of `resource` object (in the above case, `field` and `where`) are taken from the chain function names of the [squel](https://www.npmjs.com/package/squel). There are more. Refer their docs and use them accordingly.
+
+> When used with TypeScript, you should mark type of `resource` with the `import`ed `Resource` class.
+> Like `const resource:Resource = {...}`.
#### Step 3: Sqlify
+
```js
-...
+// ...
sqlify(chain, resource);
-...
+// ...
```
`sqlify` function wont return anything. It simply do things in in-place.
-#### Step 4: Watch stuff...
+#### Step 4: Watch stuff
+
```js
-...
+// ...
// parse query
-let query = chain.toString();
+const query = chain.toString();
// see it
-lme.s(query);
+console.log(query);
// => SELECT name, age, address FROM users WHERE (name='Swa') AND (age=22)
-...
+
+// ...
```
-##### Unclear about something here? Feel free to rise an issue..
+_Unclear about something here? Feel free to rise an issue.._
## Also,
-**Since `sqlify` takes in and out chain functions, you can modify it even after `sqlify`ing it.**
----
+Since `sqlify` takes in and out chain functions, you can modify it **even after** `sqlify`ing it.
+
+Example:
+
+```js
+// ...
+
+const chain = squel.select().from('users');
-> :green_heart: Find some time to contribute :star: to accommodate other functionalities from [squel](https://www.npmjs.com/package/squel).
+sqlify(chain, resource);
+
+chain.limit(10);
+
+chain.toString(); // Voila!
+```
+
+### Supported Squel Functions
+
+The following fields can be used inside the `resource` object. Logic behind the usage of these functions can be found at [squel docs](https://hiddentao.com/squel).
-### supported functions
| | | | | |
-|------------|------------|------|-----------|------------|
-| cross-join | field | join | left-join | outer-join |
-| returning | right-join | set | where | group |
-| order | | | | |
+| ---------- | ---------- | ---- | --------- | ---------- |
+| `cross_join` | `field` | `join` | `left_join` | `outer_join` |
+| `returning` | `right_join` | `set` | `where` | `group` |
+| `order` | | | | |
| | | | | |
## Contributors
-- [Lakshmipriya](https://github.com/lakshmipriyamukundan)
-
+* [Lakshmipriya](https://github.com/lakshmipriyamukundan)
## v1 to v2 migration guide
-- **change the way you `require` the package:**
- - in v1, you required `sqlify` along with `squel` as:
- ```js
- const sqlify = require('sqlify');
- const squel = require('squel');
- ...
- ```
- - in v2 you've to change that code into:
- ```js
- const sqlify = require('sqlify').sqlify;
- const squel = require('sqlify').squel;
- ...
- ```
-- **change in function name:** change `fields:[]` to `field:[]` in the `resource` object.
-
*Oh yes! it's that simple.*
-
-Just in case if you liked this package, [![PayPal][badge_paypal_donate]][paypal-donations]
+* **change the way you `require` the package:**
+ * in v1, you required `sqlify` along with `squel` as:
+ ```js
+ const sqlify = require('sqlify');
+ const squel = require('squel');
+ // ...
+ ```
+ * in v2 you've to change that code into:
+ ```js
+ const { sqlify, squel } = require('sqlify');
+ // ...
+ ```
+
+* **change in function name:** change `fields:[]` to `field:[]` in the `resource` object.
+
*Oh yes! it's that simple.*
+
+ Just in case if you liked this package, [![PayPal][badge_paypal_donate]][paypal-donations]
## Change log
-- v2.3.1
- - enabling Greeenkeeper, better docs
-- v2.3.0
- - adds better error handling: (if an unsupported method is used, sqlify throws an err)
-- v2.2.0
- - adds `order` function from [squel-order](https://hiddentao.com/squel/api.html#select_order)
- - better docs
-- v2.1.1
- - adds `group` function from [squel-group](https://hiddentao.com/squel/api.html#select_group)
- - better docs
-- v2.0.0
- - fixing [#5](https://github.com/vajahath/sqlify/issues/5) and [#2](https://github.com/vajahath/sqlify/issues/2).
- - more squel functions
-- v1.0.4
- - bug fix with 's in select queries
-- v1.0.1, 1.0.2, 1.0.3
- - bug fix (in `package.json`)
- - better docs
-
-- v1.0.0
- - initial release
+* v2.4.0
+ * TypeScript support and definitions
+ * Better docs
+* v2.3.1
+ * enabling Greeenkeeper, better docs
+* v2.3.0
+ * adds better error handling: (if an unsupported method is used, sqlify throws an err)
+* v2.2.0
+ * adds `order` function from [squel-order](https://hiddentao.com/squel/api.html#select_order)
+ * better docs
+* v2.1.1
+ * adds `group` function from [squel-group](https://hiddentao.com/squel/api.html#select_group)
+ * better docs
+* v2.0.0
+ * fixing [#5](https://github.com/vajahath/sqlify/issues/5) and [#2](https://github.com/vajahath/sqlify/issues/2).
+ * more squel functions
+* v1.0.4
+ * bug fix with 's in select queries
+* v1.0.1, 1.0.2, 1.0.3
+ * bug fix (in `package.json`)
+ * better docs
+* v1.0.0
+ * initial release
## Licence
+
MIT © [Vajahath Ahmed](https://twitter.com/vajahath7)
[badge_paypal_donate]: https://cdn.rawgit.com/vajahath/cloud-codes/a01f087f/badges/paypal_donate.svg
diff --git a/media/sqlify.png b/media/sqlify.png
new file mode 100644
index 0000000..c64ed24
Binary files /dev/null and b/media/sqlify.png differ