-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
1 parent
6f68c18
commit 44e7c0f
Showing
5 changed files
with
558 additions
and
0 deletions.
There are no files selected for viewing
Binary file not shown.
Binary file not shown.
275 changes: 275 additions & 0 deletions
275
src/app/articles/a-guide-to-creating-charts-in-react-with-typescript/page.mdx
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,275 @@ | ||
import { ArticleLayout } from '@/components/ArticleLayout'; | ||
|
||
export const article = { | ||
author: 'Bradley Dirheimer', | ||
date: '2024-10-08', | ||
title: 'A Guide to Creating Charts in React with TypeScript', | ||
description: | ||
'Visualizing data is a critical component of many modern web applications, and using charts effectively can significantly improve user experience. React, with its declarative nature, combined with TypeScript’s strong typing system, provides a robust environment for building interactive and type-safe charts. This blog post will guide you through the process of integrating charts into a React application using TypeScript.', | ||
}; | ||
|
||
export const metadata = { | ||
title: article.title, | ||
description: article.description, | ||
}; | ||
|
||
export default (props) => <ArticleLayout article={article} {...props} />; | ||
|
||
|
||
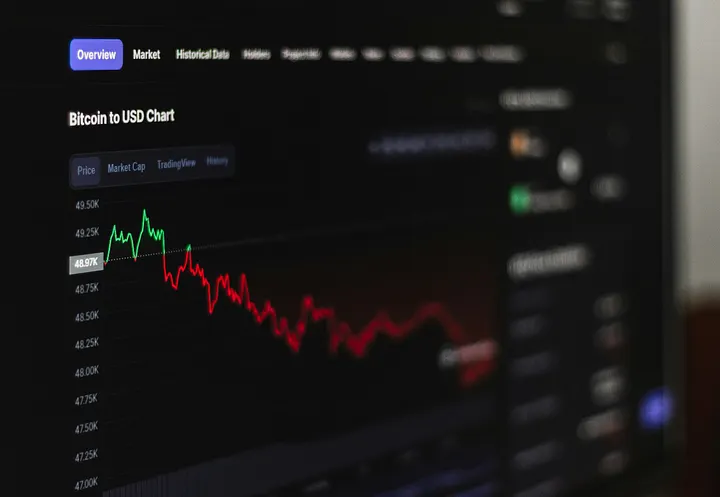 | ||
|
||
|
||
Visualizing data is a critical component of many modern web applications, and using charts effectively can significantly improve user experience. React, with its declarative nature, combined with TypeScript’s strong typing system, provides a robust environment for building interactive and type-safe charts. This blog post will guide you through the process of integrating charts into a React application using TypeScript. | ||
|
||
Why Use TypeScript with React for Charts? | ||
TypeScript helps catch potential bugs and improves the development experience by providing type safety. When creating charts, TypeScript ensures that your data structures, configurations, and event handling are well-defined, preventing common runtime errors. With React’s component-driven architecture, you can easily integrate, customize, and manage charts in your web applications. | ||
|
||
In this guide, we’ll walk through setting up charts in a React project using TypeScript and a popular charting library. | ||
|
||
Setting Up a React and TypeScript Project | ||
First, let’s set up a new React project with TypeScript: | ||
|
||
```bash | ||
npx create-react-app react-charts --template typescript | ||
``` | ||
|
||
```bash | ||
cd react-charts | ||
``` | ||
|
||
This command initializes a new React project with TypeScript as the default configuration. | ||
|
||
Installing a Charting Library | ||
There are many charting libraries available for React, such as: | ||
|
||
Chart.js: A powerful, simple, and flexible charting library. | ||
Recharts: Built on top of D3.js for React. | ||
Victory: Declarative charting library for React. | ||
For this example, we’ll use recharts as it provides an easy-to-use API and good documentation for React and TypeScript. | ||
|
||
To install Recharts: | ||
|
||
`npm install recharts` | ||
|
||
Creating a Simple Bar Chart with Recharts | ||
Now that we have our project set up and the charting library installed, let’s create a basic bar chart. | ||
|
||
Create a new component called BarChartComponent.tsx: | ||
|
||
```tsx | ||
import React from 'react'; | ||
import { BarChart, Bar, XAxis, YAxis, CartesianGrid, Tooltip, Legend, ResponsiveContainer } from 'recharts'; | ||
|
||
// Define the type for our data | ||
type ChartData = { | ||
name: string; | ||
value: number; | ||
}; | ||
|
||
// Sample data for the chart | ||
const data: ChartData[] = [ | ||
{ name: 'Page A', value: 400 }, | ||
{ name: 'Page B', value: 300 }, | ||
{ name: 'Page C', value: 200 }, | ||
{ name: 'Page D', value: 278 }, | ||
{ name: 'Page E', value: 189 }, | ||
]; | ||
|
||
const BarChartComponent: React.FC = () => { | ||
return ( | ||
<ResponsiveContainer width="100%" height={400}> | ||
<BarChart data={data} margin={{ top: 20, right: 30, left: 20, bottom: 5 }}> | ||
<CartesianGrid strokeDasharray="3 3" /> | ||
<XAxis dataKey="name" /> | ||
<YAxis /> | ||
<Tooltip /> | ||
<Legend /> | ||
<Bar dataKey="value" fill="#8884d8" /> | ||
</BarChart> | ||
</ResponsiveContainer> | ||
); | ||
}; | ||
|
||
export default BarChartComponent; | ||
``` | ||
|
||
In this example: | ||
|
||
We define a ChartData type, ensuring that every data point has a name (string) and a value (number). | ||
We create a simple bar chart using Recharts’ BarChart component. We configure the chart with axes, a tooltip, and a legend, and make the chart responsive using ResponsiveContainer. | ||
The Bar component renders the bars, and we pass the dataKey as value to tell the chart where to pull the numerical values from. | ||
Use the BarChartComponent in your app: | ||
Open src/App.tsx and add the new chart component. | ||
|
||
```tsx | ||
import React from 'react'; | ||
import './App.css'; | ||
import BarChartComponent from './BarChartComponent'; | ||
|
||
function App() { | ||
return ( | ||
<div className="App"> | ||
<h1>React Chart Example</h1> | ||
<BarChartComponent /> | ||
</div> | ||
); | ||
} | ||
|
||
export default App; | ||
``` | ||
|
||
> Now, run your application: | ||
```bash | ||
npm start | ||
``` | ||
|
||
You should see a bar chart displayed on the screen with sample data. This is a simple example, but the structure allows you to scale easily and add other chart types or more complex configurations. | ||
|
||
Adding Type Safety for Chart Props | ||
Let’s take this a step further by making our chart component reusable with type-safe props. | ||
|
||
Update BarChartComponent.tsx to accept props: | ||
|
||
```tsx | ||
type BarChartProps = { | ||
data: ChartData[]; | ||
xAxisKey: string; | ||
yAxisKey: string; | ||
}; | ||
|
||
const BarChartComponent: React.FC<BarChartProps> = ({ data, xAxisKey, yAxisKey }) => { | ||
return ( | ||
<ResponsiveContainer width="100%" height={400}> | ||
<BarChart data={data} margin={{ top: 20, right: 30, left: 20, bottom: 5 }}> | ||
<CartesianGrid strokeDasharray="3 3" /> | ||
<XAxis dataKey={xAxisKey} /> | ||
<YAxis /> | ||
<Tooltip /> | ||
<Legend /> | ||
<Bar dataKey={yAxisKey} fill="#8884d8" /> | ||
</BarChart> | ||
</ResponsiveContainer> | ||
); | ||
}; | ||
``` | ||
|
||
Now, the BarChartComponent accepts props for data, xAxisKey, and yAxisKey, which allow you to customize which properties to map to the X and Y axes. This type-safe structure ensures that any data you pass must conform to the ChartData type, preventing mismatches and errors. | ||
|
||
You can now use the component like this: | ||
|
||
`<BarChartComponent data={data} xAxisKey="name" yAxisKey="value" />` | ||
|
||
Advanced Charts and Customization | ||
Recharts offers a variety of charts such as line charts, pie charts, and area charts, all of which you can configure with TypeScript. Here’s an example of a Line Chart with custom tooltips: | ||
|
||
```tsx | ||
import { LineChart, Line, XAxis, YAxis, CartesianGrid, Tooltip, Legend, ResponsiveContainer } from 'recharts'; | ||
|
||
const LineChartComponent: React.FC<BarChartProps> = ({ data, xAxisKey, yAxisKey }) => { | ||
return ( | ||
<ResponsiveContainer width="100%" height={400}> | ||
<LineChart data={data} margin={{ top: 20, right: 30, left: 20, bottom: 5 }}> | ||
<CartesianGrid strokeDasharray="3 3" /> | ||
<XAxis dataKey={xAxisKey} /> | ||
<YAxis /> | ||
<Tooltip contentStyle={{ backgroundColor: '#ccc' }} /> | ||
<Legend /> | ||
<Line type="monotone" dataKey={yAxisKey} stroke="#8884d8" activeDot={{ r: 8 }} /> | ||
</LineChart> | ||
</ResponsiveContainer> | ||
); | ||
}; | ||
``` | ||
|
||
Handling Asynchronous Data | ||
Many real-world scenarios involve fetching data from an API and rendering it in charts. You can handle asynchronous data in TypeScript with tools like fetch or libraries like axios: | ||
|
||
```tsx | ||
import { useEffect, useState } from 'react'; | ||
import axios from 'axios'; | ||
|
||
const DataFetchingChart: React.FC = () => { | ||
const [data, setData] = useState<ChartData[]>([]); | ||
|
||
useEffect(() => { | ||
axios.get('/api/data').then((response) => { | ||
setData(response.data); | ||
}); | ||
}, []); | ||
|
||
return <BarChartComponent data={data} xAxisKey="name" yAxisKey="value" />; | ||
}; | ||
``` | ||
|
||
This example demonstrates fetching data from an API and updating the chart once the data has been retrieved. | ||
|
||
## Conclusion | ||
|
||
Creating charts in React with TypeScript offers both flexibility and type safety, making it easier to handle data, manage props, and ensure that your chart components work as expected. With libraries like Recharts, you can quickly build a variety of charts, while TypeScript’s strong typing helps prevent bugs. | ||
|
||
To sum up: | ||
|
||
Choose a charting library that fits your project needs. | ||
Leverage TypeScript to define clear types for your chart data and props. | ||
Handle asynchronous data by integrating APIs and React’s lifecycle methods. | ||
Customize charts based on your application’s requirements for interactivity and design. | ||
With this approach, you’ll have a solid foundation for creating robust and scalable data visualizations in React using TypeScript. | ||
|
||
## API for rechart | ||
|
||
`Charts` | ||
- AreaChart | ||
- BarChart | ||
- LineChart | ||
- ComposedChart | ||
- PieChart | ||
- RadarChart | ||
- RadialBarChart | ||
- ScatterChart | ||
- FunnelChart | ||
- Treemap | ||
- SankeyChart | ||
|
||
`General Components` | ||
- ResponsiveContainer | ||
- Legend | ||
- Tooltip | ||
- Cell | ||
- Text | ||
- Label | ||
- LabelList | ||
- Customized | ||
|
||
`Cartesian Components` | ||
- Area | ||
- Bar | ||
- Line | ||
- Scatter | ||
- XAxis | ||
- YAxis | ||
- ZAxis | ||
- Brush | ||
- CartesianAxis | ||
- CartesianGrid | ||
- ReferenceLine | ||
- ReferenceDot | ||
- ReferenceArea | ||
- ErrorBar | ||
- Funnel | ||
|
||
`Polar Components` | ||
- Pie | ||
- Radar | ||
- RadialBar | ||
- PolarAngleAxis | ||
- PolarGrid | ||
- PolarRadiusAxis | ||
|
||
`Shapes` | ||
- Cross | ||
- Curve | ||
- Dot | ||
- Polygon | ||
- Rectangle | ||
- Sector |
Oops, something went wrong.